PlantUML
This is an open source project that allows you to quickly draw diagrams like the one below in text.
Sequence diagram
Usecase diagram
Class diagram
Activity diagram
Component diagram
State diagram
Object diagram
Install PlantUML from the Visual Studio Marketplace.
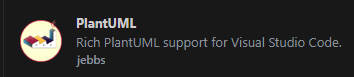
PlantUML handles files in the *.wsd, *.pu, *.puml, *.plantuml, and *.iuml formats as UML diagram files, so please use these extensions.
Once you have written the text that will become the base of your UML diagram, press Alt + D. You can preview the UML diagram as shown below.
Example
@startuml
title test
class class1 {
method1()
}
class class2 {
method2()
}
class1 --|> class2
@enduml
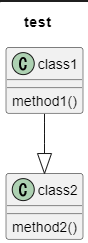